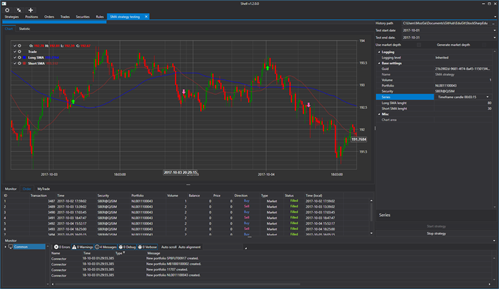
In this paper, I will show how to use graphic components included in S#.API, in order to create a fully-featured application of the
S#.Shell:
You will learn how to make a professional-level program with connection settings, output of securities, prices, and charts (and all this is saved and loaded upon restart). And the complexity of creating such an application is not a few months, but just a few hours (it is no joke! read to the end). This is the main advantage of the graphic framework, which I called by analogy S#.UI (this name is unofficial, I think it up myself).
I will not use complex constructs and design patterns that are understandable only to professional programmers. On the contrary, the paper's purpose is to show that the learning curve on creating your trading applications using S#.API is very short.
You will also be interested if you work in a company and make your own unique software (for example, you work in proprietary trading or a brokerage company). In this paper, you will be able to learn the practice of creating such systems (especially if you have just taken up your duties).
What You Need
1) Visual Studio 2017 (Community, free version), in which we will program.
2) Free connection to test exchange trading, I will use QUIK.
Creating Project
We create a new WPF application in Visual Studio.
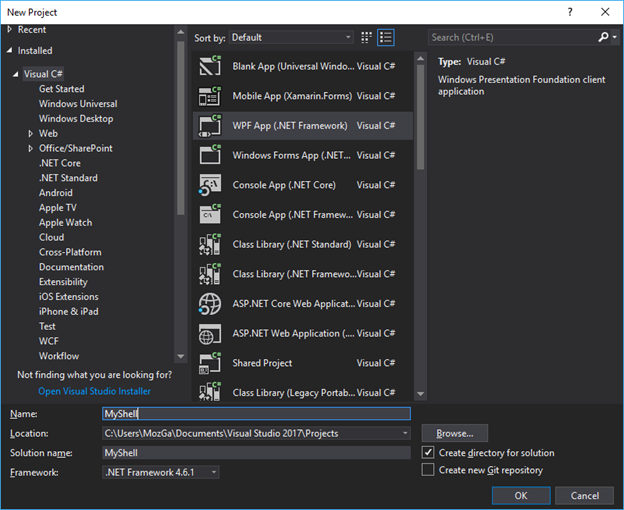
After that we need to add the S#.API libraries, and how to do this is described
here. I prefer installing with Nuget.
Since all S#.API graphic elements are based on DevExpress, and DevExpress libraries are included in S#.API, it would be foolish not to use them. All information about DevExpress graphic elements can be found in Google.
Let's go to the MainWindow.xaml window editor.
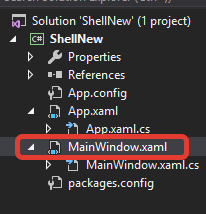
We replace Window with DXWindow, we will need it to use different color schemes.
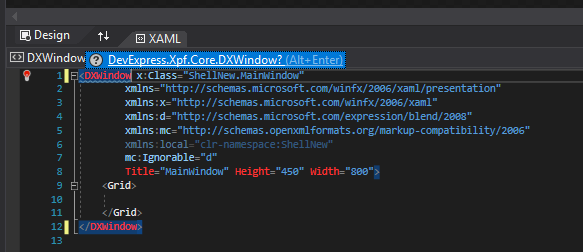
Visual Studio will prompt us to add the necessary libraries.
We split the window into three parts, there will be a bar with buttons of connection setting and connection at the top. The window with logs will be below. All other panels are in the middle. The easiest way is to split the window in this way using LayoutControl from DevExpress.
We will add the elements we need to the resulting three parts.
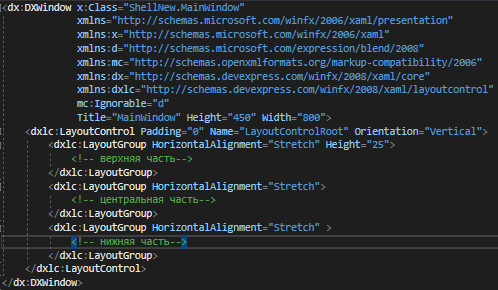
Configuring Connection to Connector
We add two buttons, one button to configure connection and the second button to connect. To do this, we use the SimpleButton button from DevExpress. The buttons will be located at the top of application. We will place pictures familiar with Terminal and Designer on each button.
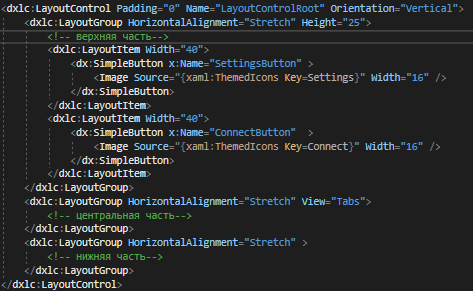
We will see such an image in the upper right corner of the screen form.
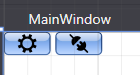
We double-click each button to create button click event handlers.
In MainWindow code it is necessary to declare the connector, as well as the file location and name where the connector settings will be stored.

We will open the connector configuration window in the connector settings button click event handler and save it to a file.

We will check in the constructor whether there is a directory and a file with the connector settings and, if there is one, we will load it into the connector.
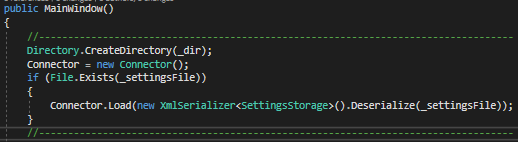
Most S#.API objects have Save and Load methods, with which it is possible to save and load this object from an XML file.
We connect the connector in the connection button click event handler method.

Now we can run the program and test it.
Setting Dark Theme
I prefer a dark theme. Therefore, we make the program theme dark at once. To do this in the App.xaml file
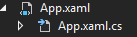
We replace Application with charting:ExtendedBaseApplication. Visual Studio will prompt us to add the necessary libraries. And in the file App.xaml.cs delete ": Application". The code will be the following.
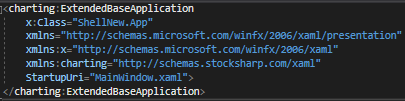
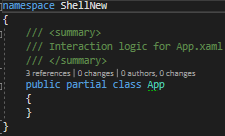
We write ApplicationThemeHelper.ApplicationThemeName = Theme.VS2017DarkName in the MainWindow constructor;
Full code at the moment:
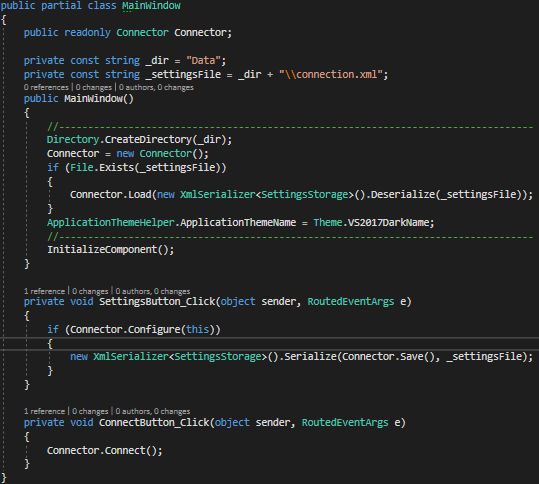
Run to check the dark theme.
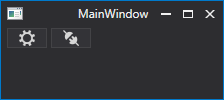
Creating Security Panel
We add the XAML folder where we will store all the created controls.
We add our first UserControll to the folder, let's call it SecurityGridControl.
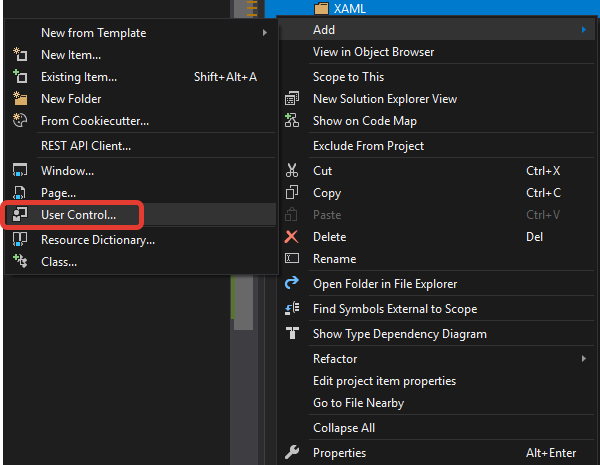
We add one SecurityPicker element to it. In which the available securities will be displayed. Similar to the main window, we will use LayoutControl from DevExpress.
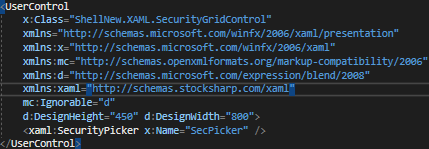
We go to the main window constructor and change the central part to the tab view. We place created control with SecurityPicker in one of the tabs.
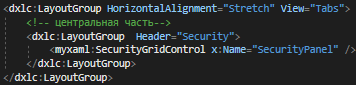
Now we have the security panel, we need to set it a data source, in our case it is a connector. It was possible to write SecurityPanel.SecPicker.SecurityProvider = Connector in MainWindow constructor.
But we will not place code in the MainWindow that is not related to it. Therefore, I will create the Instance static variable, and assign it the MainWindow value in the MainWindow constructor.
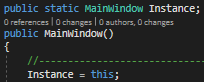
Now we can call the MainWindow properties anywhere in our program using MainWindow.Instance.XXX code.
In the SecurityGridControl constructor, we specify Connector as a data source.

We run the program to check.
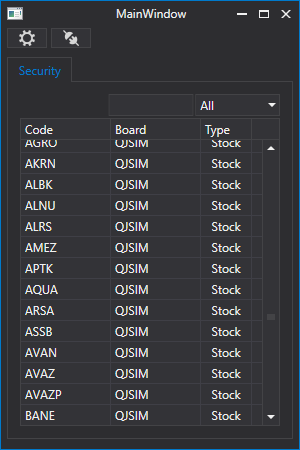
Adding Logging
The operation of the program, connector or robot must be monitored. For this purpose, S#.API has a special LogManager class. This class receives messages from sources and sends them to listeners. In our case, the sources will be Connector, strategies, etc., and listeners will be a file and log panel.
In the MainWindow code, we declare the LogManager object and the location where it will be stored.

In the MainWindow constructor, we create LogManager, set in it Connector as the source, and a file as the listener.
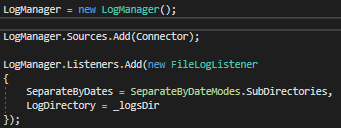
Similar to the security panel, we create a log panel, add another UserControl to the XAML folder. We call it MonitorControl. We add the Monitor element to it.
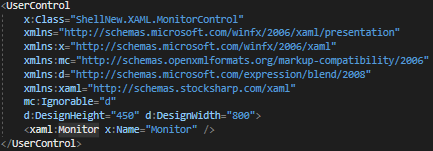
In the MonitorControl constructor, we set Monitor as a listener in LogManager.
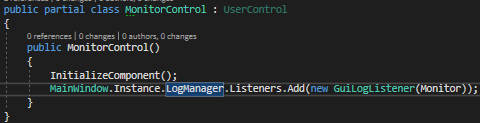
We add the created MonitorControl to the bottom of MainWindow.

We run the program to check.
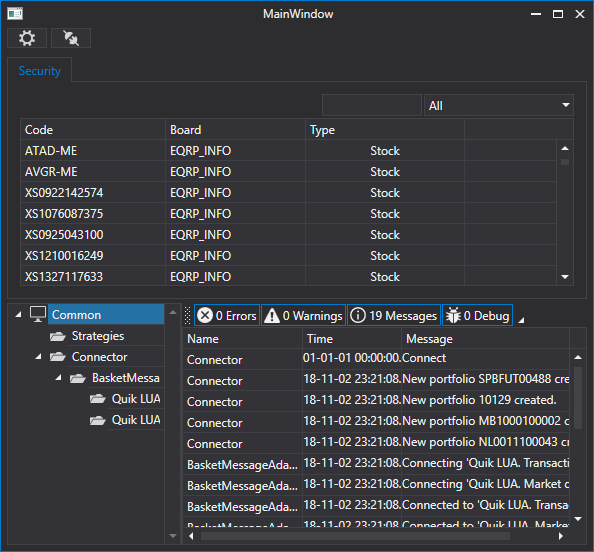
Creating Portfolio Panel
Similar to the security panel, we create a log panel, add another UserControl to the XAML folder. We call it PortfolioGridControl. We add the PortfolioGrid element to it.
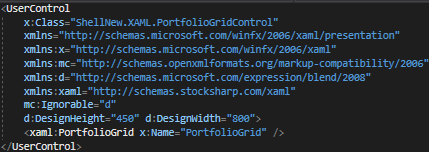
In PortfolioGridControl constructor, we need to subscribe to new portfolio appearance events and event of new position appearance at Connector.
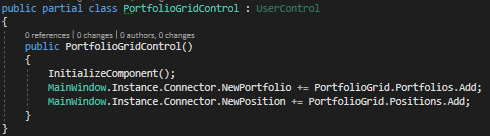
Thus, when a new portfolio appears, it will be displayed in the portfolio panel, and when a new position appears in the portfolio panel, the portfolio will update the position.
We add the created PortfolioGridControl panel to the central part of MainWindow.
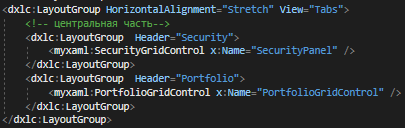
We run the program to check.
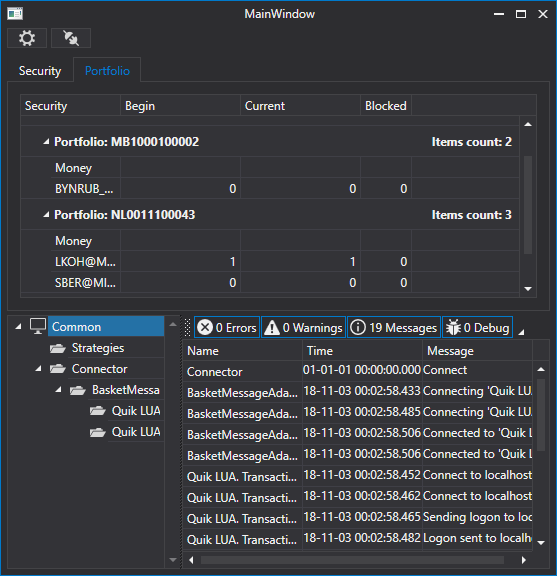
We have a tab with portfolios.
Creating Order Panel
The order panel in S#.API has the ability to register orders, cancel orders and reregister them.
Similar to the security panel, we create an order panel, add another UserControl to the XAML folder. We call it OrderGridControl. We add the OrderGrid element to it.
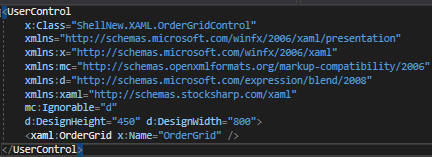
OrderGrid has the OrderRegistering event, the OrderReRegistering event, and the OrderCanceling event.
Let's create their handlers.
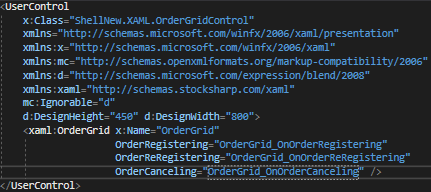
In the order registration event handler, we create an OrderWindow window where we need to specify data sources for securities, portfolios, and market data. For all cases, this will be the Connector.
After that, we call OrderWindow using ShowModal method, if the OK button was clicked in this window, we register the order via the connector using the RegisterOrder method.
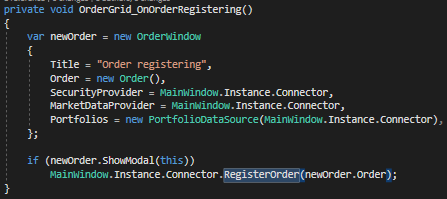
We do the same in the order reregistration event handler. Only in this case the event receives the Order object - this is the order that needs to be reregistered. Therefore, in OrderWindow, we specify Order = order.ReRegisterClone(newVolume: order.Balance) to fill in the fields of the OrderWindow window.
After that, we call OrderWindow using ShowModal method, if the OK button was clicked in this window, we will reregister the order via connector using the ReRegisterClone method. We pass to it old order, which should be cancelled, and the new one, which should be registered.
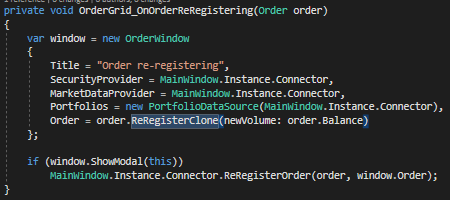
In the order canceling event handler, it is enough to call the CancelOrder method and pass to it the order that need to be canceled.

In order for Orders to be displayed in the OrderGrid, it is necessary in the OrderGridControl constructor to subscribe to new order appearance events and to a registration error event and pass these events to the OrderGrid.
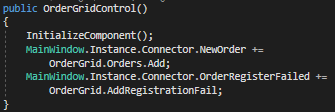
We add the created OrderGridControl panel to the central part of MainWindow.
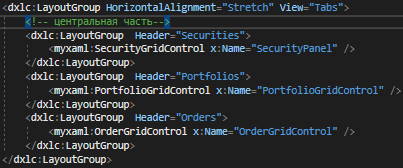
We run the program to check.
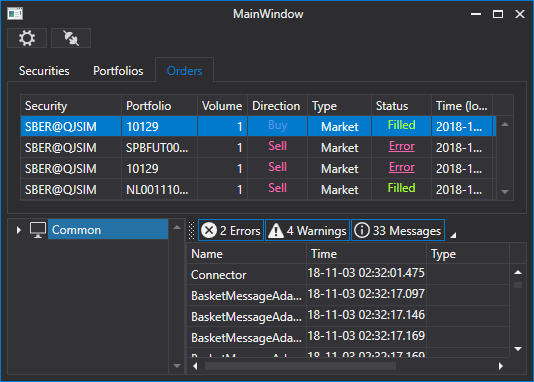
Creating Panel of Own Trades
Similar to the security panel, we create a panel of own trades, add another UserControl to the XAML folder. We call it MyTradeGridControl. We add the MyTradeGrid element to it.
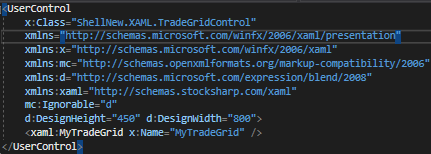
In the MyTradeGridControl constructor, we need to subscribe to the new own trade appearance events and pass it to MyTradeGrid.

We add the created OrderGridControl panel to the central part of MainWindow.
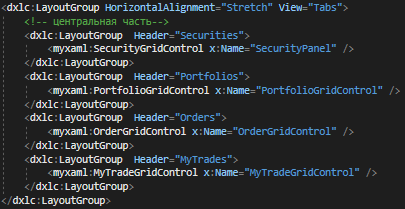
We run the program to check.
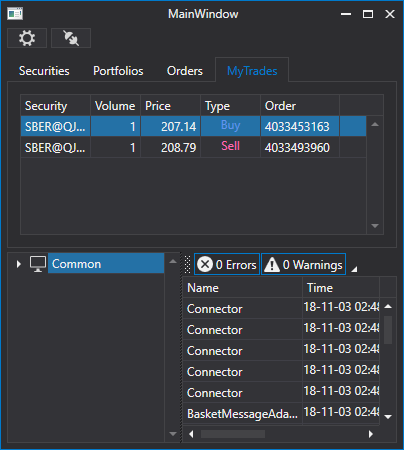
Creating Order Book Panel
Similar to the previous panels, we create an order book panel, add another UserControl to the XAML folder. We call it MarketDepthControl.
In MainWindow we have already used LayoutControl, in this control we will also use LayoutControl. We split the panel into two parts horizontally.
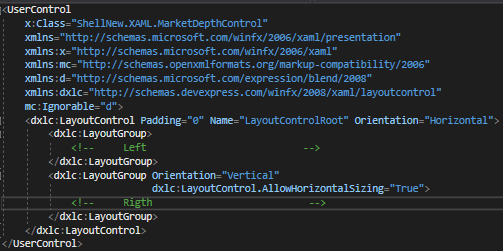
We add SecurityPicker to the left side, we use it when we created the security panel.

Let's split the right part into vertical parts. There will be an order book on the top right.

In MarketDepthControl, we need to set some MaxHeight value, otherwise the application will not start.
Below the order book we will place the elements of specifying a portfolio, price, and order volume.
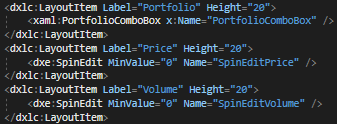
Here it is worth noting the Label property of LayoutItem, it allows to set the text over the element. And also the SpinEdit element from DevExpress, in which it is convenient to set numerical values. These elements look like this.
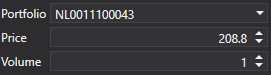
Even below, we will place the buttons to buy, sell.
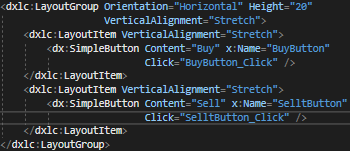
Full code.
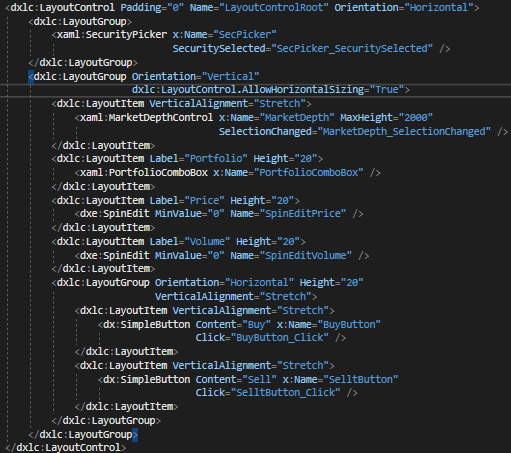
In the MarketDepthControl constructor, we set the security source for SecurityPicker and the portfolio source for PortfolioComboBox, in our case it will be Connector.
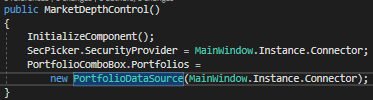
We create a security selection event handler in SecurityPicker. We check in it whether the selected security is not equal to zero. If it is not equal to zero, we save the received security to a local variable, we will need it when updating the order book. After that, we clear and register the received security in Connector to receive an order book using the RegisterMarketDepth method. Using the GetMarketDepth method, we get the current order book on security to update the MarketDepthControl.
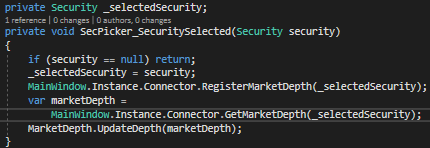
To keep the order book updated in the MarketDepthControl constructor, we will subscribe to the MarketDepthChanged order book change event at the connector. In the handler of this event, we will check which security the received order book belongs to, and if it belongs to the security selected in SecurityPicker, we update MarketDepthControl with it.
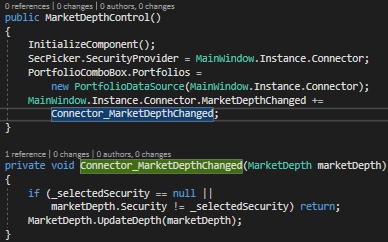
We add the created MarketDepthControl panel to the central part of MainWindow.

At this stage, we can run the program and check the order book update operation.
We create a buy and sell buttons click event handler. In each handler we create an Order, in which we specify the security selected in SecurityPicker, the portfolio selected in PortfolioComboBox, the volume and price from the corresponding SpinEdit. We register the order in Connector using the RegisterOrder method.
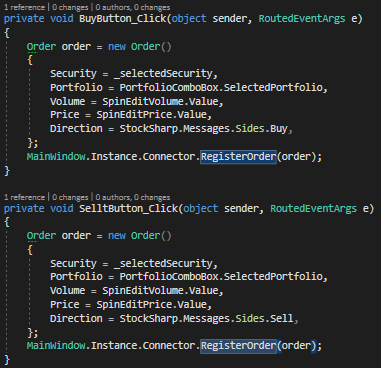
Both handlers differ only by the order direction.
Let's make that the SpinEditPrice value is changed to the selected quote price when selecting a quote in the order book. To do this, we will create a handler for the SelectionChanged event at MarketDepthControl. In which we will update SpinEditPrice value by the price of the selected quote if the selected quote is not equal to zero
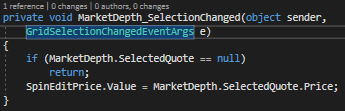
We run the program to check.
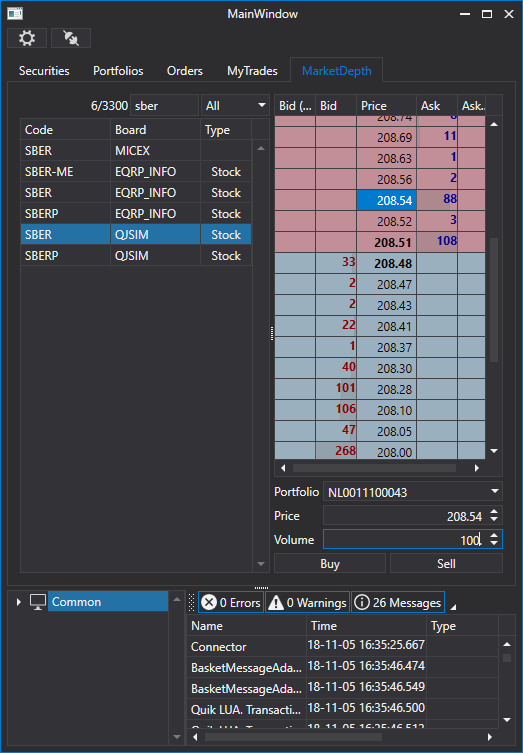
Saving Market Data
To save portfolios, securities, platforms, we need the CsvEntityRegistry class. We need to pass the entities storage location to it and call the Init method to load them.

To save candles, trades, etc., we will need StorageRegistry.

We will also need the SnapshotRegistry snapshot storages registry.

All this we pass to Connector when it is created.

Here I also specified that Connector will reconnect when the connection is broken, and also specified how many history days should be downloaded.
The Connector.LookupAll(); string queries the available data.
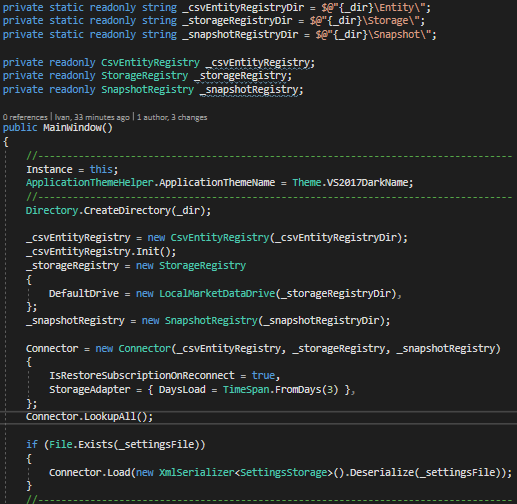
After starting the application, we will see that new folders have appeared in the Date folder.
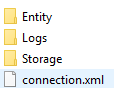
And when reconnecting the security and portfolio panels will already be filled.
Creating Strategy Panel
I will create the strategy panel just like all previous panels.
We add another UserControl to the XAML folder. We call it StrategyControl. We split the screen form into two parts using the LayoutControl.
There will be a tab with a candle chart on the left side.

As well as a tab with strategy statistics,
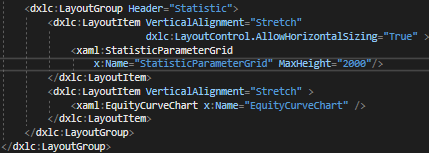
Here I use StatisticParameterGrid to display strategy statistics and EquityCurveChart to display profit and loss chart.
In StatisticParameterGrid, we need to set some MaxHeight value, otherwise the application will not start.
The strategy properties in PropertyGridEx will be configured on the right side.

As well as strategy start and stop buttons.
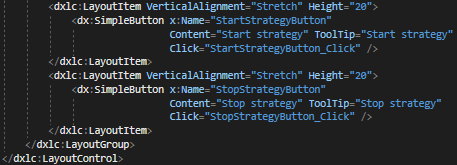
Full code.
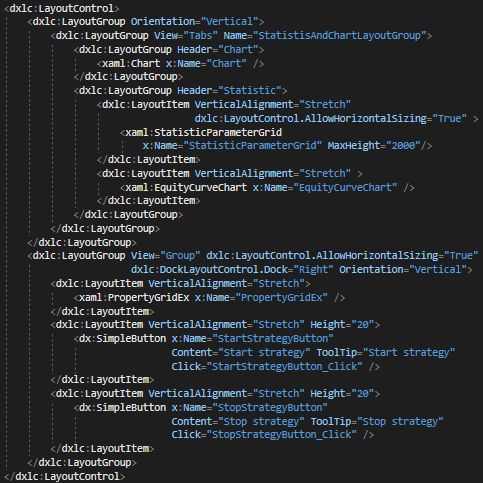
In the StrategyControl constructor, we set Connector as a data source for PropertyGridEx, we carried out similar actions in almost every control.
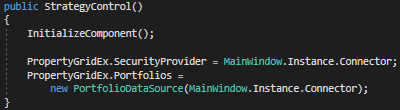
We need to somehow pass the strategy to our control. To do this, in StrategyControl I will create a BindStraregy method that will receive a strategy, save a link to it in the local variable, and also set a strategy in PropertyGridEx and StatisticParameterGrid.
Using the SetChart method, we pass the Chart candle chart to the strategy, after that, the Chart can be obtained in the strategy using the GetChart method. We also set the Connector for the strategy.
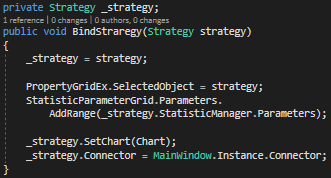
When working with the profit and loss chart, it is necessary to take into account that the strategy may start and stop several times, so every time the strategy starts, the chart should be cleared. To do this, let's create the ResetEquityCurveChart method, in which we will first clear EquityCurveChart. After that we need to create graphic elements for EquityCurveChart, for them we can set a name, color and line type.
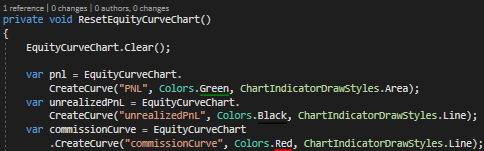
Then, we subscribe to the PnL change event of the strategy and in this event handler we draw a new value on the EquityCurveChart profit loss chart.
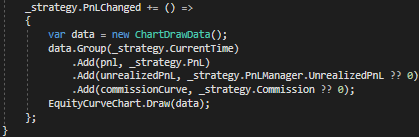
Full method code.
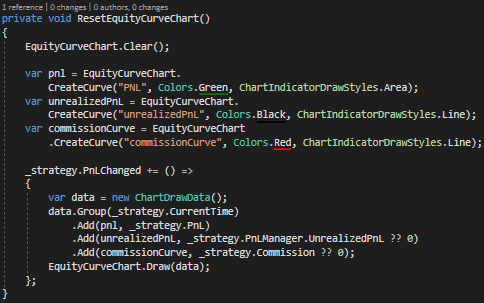
We will call this method in the Start button click event handler. And also we will reset the strategy state and run it.
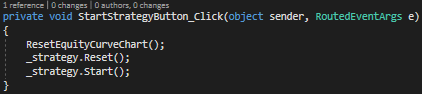
We will stop the strategy in the Stop button click event handler.

We add the created StrategyControl panel to the central part of MainWindow.

Creating Strategy
For example, let's consider creating a simple strategy with candles. Which will buy if the candle is growing (green), and sell if the candle is falling (red).
Let's create another folder in the project and store all our strategies in it. We create a new class in this folder and call it SimpleStrategy. All S# strategies must be inherited from the base class Strategy.
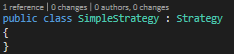
Since our strategy uses candles, we will create a public property CandleSeries, and set its default value in our strategy constructor.
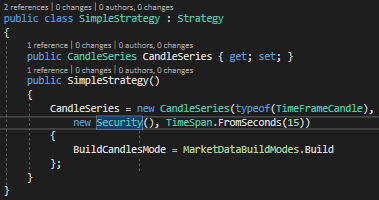
Here I specified that candles in CandleSeries will be TimeFrameCandle with time span of 15 seconds (TimeSpan.FromSeconds(15)). We can specify the candle creating mode for CandleSeries - BuildCandlesMode. I specified that candles will be built (MarketDataBuildModes.Build), by default they will be built from ticks, but we can specify other data types as well.
Since we made the CandleSeries a public property, the CandleSeries can be additionally configured from the PropertyGridEx described in the previous paragraph.
All strategies have methods that can be overridden, we will need to override the OnStarted method. Which is called before the launch of the strategy and allows to preset its starting state.
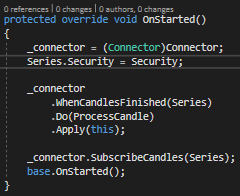
Here we set the security for CandleSeries, which is specified in the PropertyGridEx. After that, we create a rule for processing the completed candle. See the documentation for details on working with rules. In the rule we specify the method that will process each completed candle, in our case it is the ProcessCandle method, it will be described later. After everything is set, we subscribe to the appearance of candles on the CandleSeries in connector using the SubscribeCandles method.
In our case, the ProcessCandle method contains the main strategy logic.
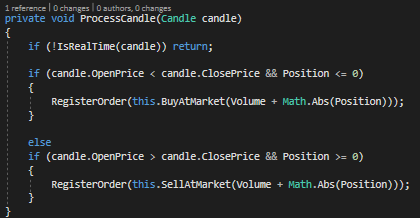
First of all, we need to determine whether the candle is real-time or historical, if the candle is historical, we ignore it. Not all strategies require this, for example, for strategies based on order books, this is not required, since order books are always real-time. There is no general way to determine whether a candle is real-time or historical, and in each strategy this problem will have to be solved independently depending on the strategy requirements. In this case, I will simply compare the candle closing time with the connector time, and if it does not exceed a certain lag, then I consider the candle real-time.

Next, we consider what candle it is and what the strategy current position. If the candle is growing, then at a position equal to 0, we will open a position with a market order for the volume set by us in PropertyGridEx. If the candle is growing and the position is less than 0, we reverse the position.

We do opposite actions for a falling candle.

At the moment, our strategy is ready to work. It should be passed to SimpleStrategyControl, which we created in the previous paragraph using the BindStraregy method. We do this in the MainWindow constructor immediately after the MainWindow components are initialized.

We run the program to check.
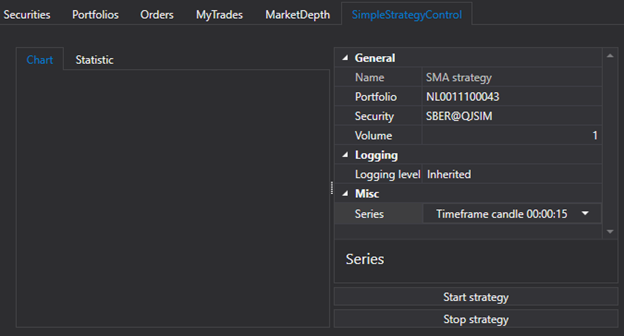
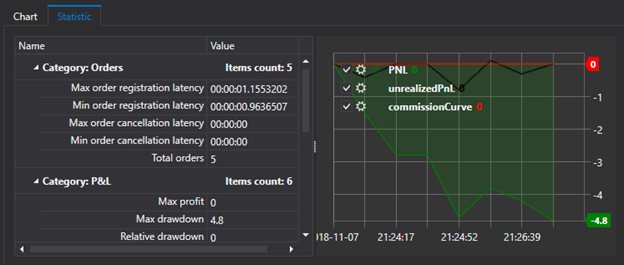
The strategy works, trades are made, but there are no candles and trades on the chart yet.
Adding Candles and Trades to Chart from Strategy
In the paragraph about the strategy panel, we passed the Chart candle chart to the strategy using the SetChart method. In the OnStarted strategy method, we check whether the strategy has a chart set, and if it is set, then we initialize the chart, as well as subscribe to the events of new own trade appearance and candle change.
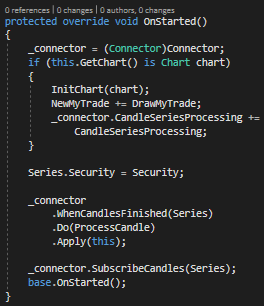
InitChart chart initialization method.
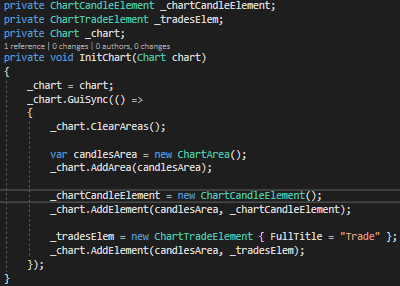
Here we save the link to Chart in a local variable. We clear the chart. We also create chart elements for candles and trades and pass them to the chart.
The chart.GuiSync(()=>{ ... }); construct is necessary in order to initialize the chart in the main thread.
CandleSeriesProcessing is a method for drawing candles on a chart.
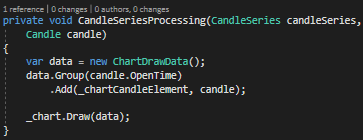
Here we get a candle from the CandleSeriesProcessing event of the connector, create ChartDrawData to display it on the chart. We specify the time data.Group(candle.OpenTime), specify that the candle should be added to the candle element of the chart .Add(_chartCandleElement, candle);. And we specify that the chart should draw new data.
We perform similar actions for trades.
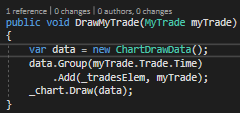
We run the program to check.
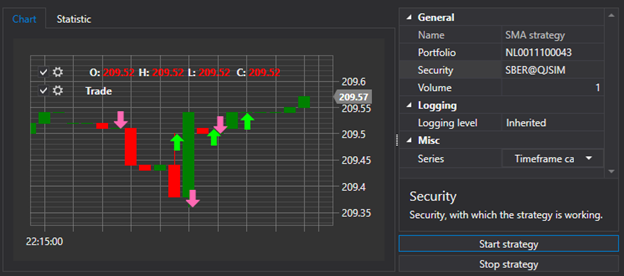
Short conclusion
It is not necessary to spend a lot of time to create a complex and professional-looking application. In a few hours we created the fully-featured application with the ability to configure, display and trade directly.
Do not be afraid to try and create your own programs. I hope this paper will help you get used to this business.